What is GRPC
Understanding what RPC means
Remote Procedure Call (RPC) is a protocol that one program can use to request a service from a program located in another computer on a network without having to understand the network’s details. RPC is used to call other processes on the remote systems like a local system. A procedure call is also sometimes known as a function call or a subroutine call.
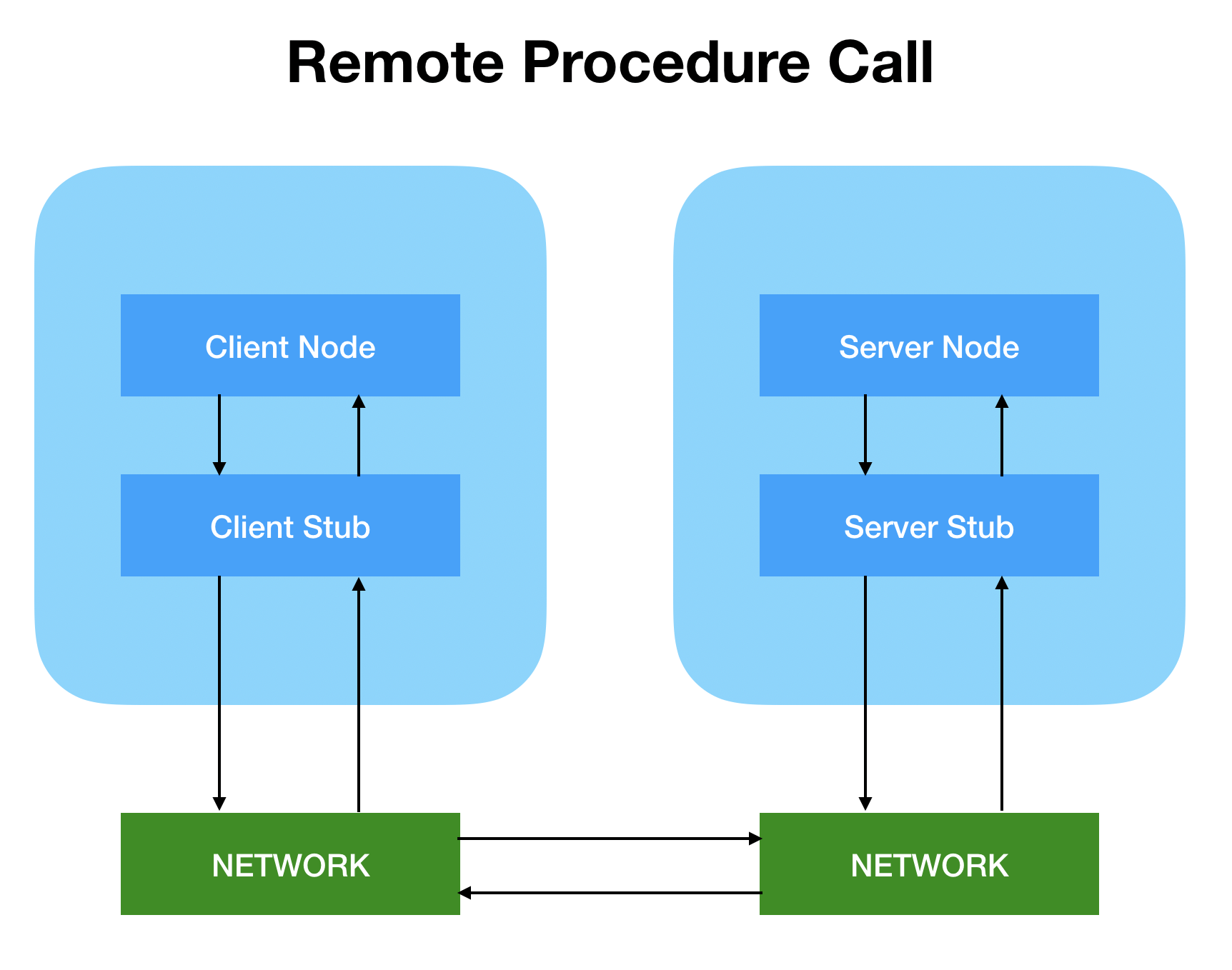
How GRPC comes into play
Before diving into the meaning and uses of GRPC, I’ll start by explaining the problem GRPC solves. GRPC was created to solve the problem of communication and communicating effectively. Let’s use an example of two applications expected to carry out a simple task of sending and receiving messages. Both applications use the same language and the communication medium is essentially the same shared computer. For now, we will keep it simple and make the assumption that communication is unidirectional (communication happens in one way at a time).
- The Sender sends a message (request) to the Receiver and waits for a response
- The Receiver receives the message (request) and returns a response as another message
Because the 2 applications are on the same computer speaking the same language, the communication is very simple. There is no need for anything special to enable successful communication.

Now let’s change the context, imagine that we have 2 applications that are in separate computers, and use different languages. For this example, for successful communication to occur, we will need a mechanism to enable distributed communication. The mechanism will need to define some form of protocol (agreed-upon rules) and will also need to do some form of encoding and decoding of messages sent between the Sender and the Receiver. This can be seen as illustrated in the diagram below.
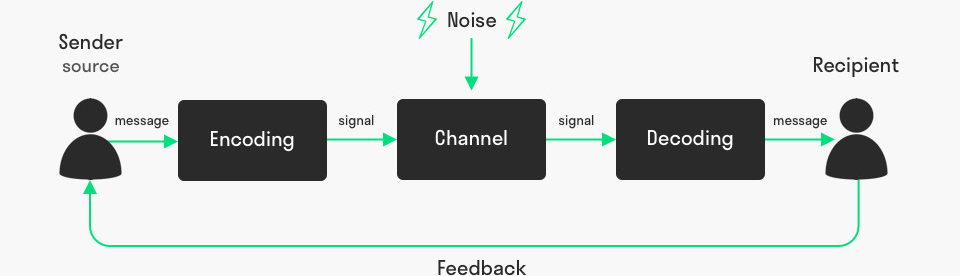
GRPC was developed by Google as an open-source evolution of their internal RPC technology named Stubby, and they continue to be the stewards of the official open-source project.
gRPC is a modern open-source high-performance RPC framework that can run in any environment. It can efficiently connect services in and across data centers with support you can plug in for load balancing, tracing, health checking, and authentication. It is also applicable in the last mile of distributed computing to connect devices, mobile applications, and browsers to back-end services.
Google has been using a single general-purpose RPC infrastructure called Stubby to connect the large number of micro-services running within and across their data centers for over a decade. Their internal systems have long embraced the microservice architecture gaining popularity today. Stubby has powered all of Google’s micro-services interconnect for over a decade and is the RPC backbone behind every Google service that you use today. In March 2015, they decided to build the next version of Stubby in the open so they can share what they learned with the industry and collaborate with them to build the next version of Stubby both for micro-services inside and outside Google but again, also for the last mile of computing (mobile, web, and IoT).
Interesting facts
- What Does the g in gRPC Stand For (https://grpc.github.io/grpc/core/md_doc_g_stands_for.html)
- Client and Server communicate over stud which talks to the underlying grpc framework
- gRPC is a Cloud Native Computing Foundation incubating project
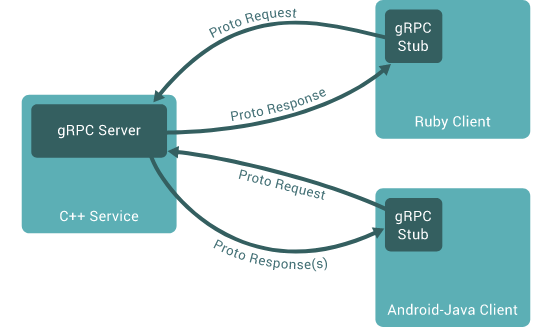
What is Protocol Buffer
Protocol buffers are Google’s language-neutral, platform-neutral, extensible mechanism for serializing structured data. gRPC uses protobuf as a language to define data structures and services. You can compare this with a strict documentation for REST services. The Protobuf syntax is very strict so that the machine can compile.
For more information:
- Protocol Buffers (https://developers.google.com/protocol-buffers/docs/overview) - The official Google developer guide
- Working with Protocol Buffers (https://grpc.io/docs/guides/#working-with-protocol-buffers) - Protocol buffers explained on gRPC website
Interesting facts
- Other Options: Google flat buffers, Microsoft Bond
- GRPC uses HTTP/2 as its transfer protocol(Binary framing, Multiplexing, Server Push)
Types Of GRPC
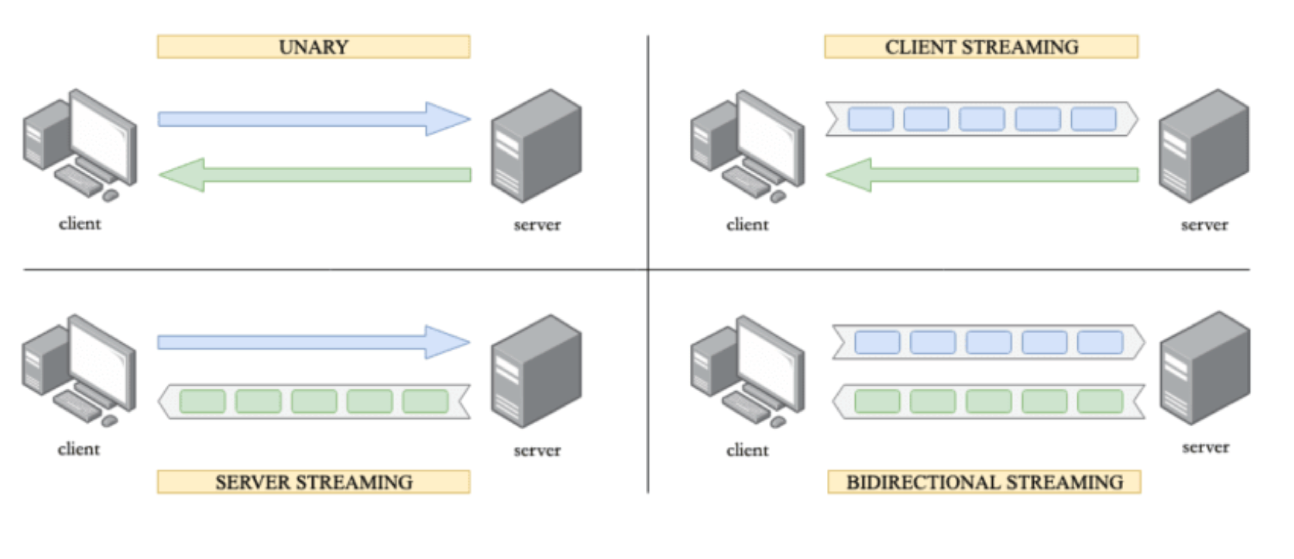
gRPC lets you define four kinds of service method:
- Unary RPCs where the client sends a single request to the server and gets a single response back, just like a normal function call. rpc SayHello(HelloRequest) returns (HelloResponse);
- Server streaming RPCs where the client sends a request to the server and gets a stream to read a sequence of messages back. rpc LotsOfReplies(HelloRequest) returns (stream HelloResponse);
- Client streaming RPCs where the client writes a sequence of messages and sends them to the server, again using a provided stream. rpc LotsOfGreetings(stream HelloRequest) returns (HelloResponse);
- Bidirectional streaming RPCs where both sides send a sequence of messages using a read-write stream. rpc BidiHello(stream HelloRequest) returns (stream HelloResponse);
LET’S CODE IT!!!
Here’s a basic grpc chat communication between a server and client. The first step is to define our proto file which follows the basic structure below:
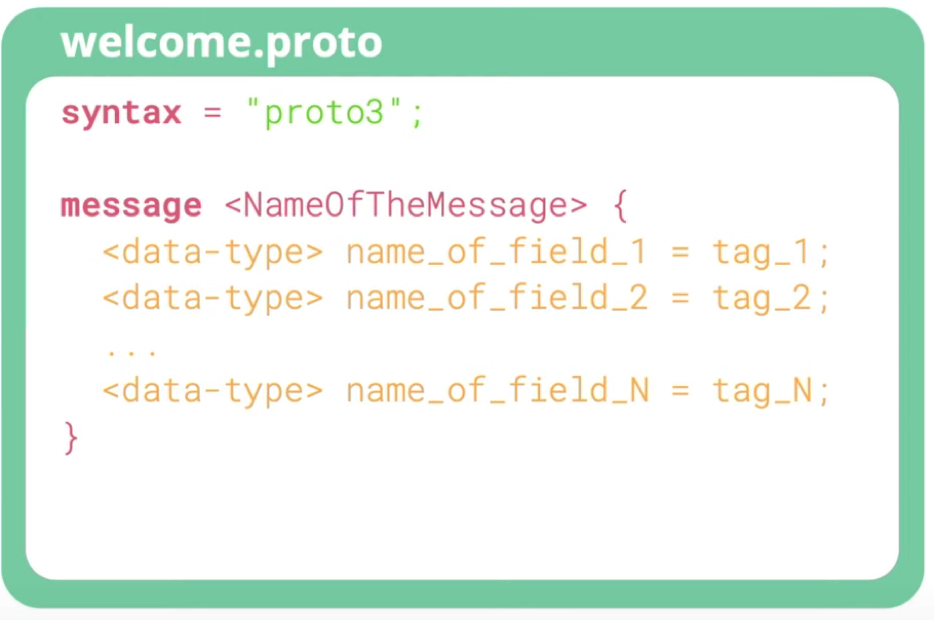
Below we have our chat.proto file:
To generate our protobuf file which would ultimately be our API contract between the client and server, we can use the command below:
Next, we can write out our grpc server where we define our methods previously declared in the chat.proto. Take a look at the SayHello method which receives a message from the client and responds back with a simple Hello From the Server!
Finally, we have a simple client which creates a new chat service client, calls the server, waits for a response, and then logs the response. Find below our sample client.go
Hurray, we have our basic application setup🥳, for our full working code visit the link below:
Keen to learn more? Get in touch
We're here to answer your questions and discuss any potential projects.